To access the contents, click the chapter and section titles.
Learn Pascal in a Three Days (2nd Ed.)
(Publisher: Wordware Publishing, Inc.)
Author(s):
ISBN: 1556225679
Publication Date: 07/01/97
Chapter 11 Pointers and Linked Lists
11-1 Dynamic Memory Allocation
The variables already used so far are called static variables. The relationship between the static variable and the memory location it refers to is established at the compilation time and does not change during the program execution. A dynamic variable, on the contrary, is created or disposed during the execution. In other words, the necessary memory location for a dynamic variable is allocated while the program is running, and may be released and allocated to another variable. In Pascal, you may create a simple dynamic variable or a complex dynamic data structure such as a linked list. A linked list may be needed in some situations when you cannot predict your memory requirements. As opposed to the linked list, the array is an example of static data structures. The memory locations associated with the array elements are allocated at the time of compilation. The disadvantage of using arrays, in such situations, is the need to allocate enough space for the maximum possible number of elements. Defining a huge array which may exceed your needs is a waste of memory; and using a small array will limit your program to a specific number of elements. The problem occurs when you need to insert a new element in the array. Dynamic data structures may expand or shrink during the program execution, and so does the associated memory. Dynamic memory allocation is accomplished by using pointers. In the following sections you learn how to declare and use pointers.
11-2 Pointers
A pointer is a special type of variable that does not hold data; instead, it holds the address of a data location. Therefore, it is said that it points to a data location. In your program, it is possible to redirect the pointer to make it point to another memory location, or to no memory location. It is also possible to release the memory associated with a specific pointer and make it available to other variables. Pointers can point to any type of data, from CHARs and INTEGERs to complex data structures such as records and linked lists.
A pointer to an integer is declared as follows:
VAR
PtrVariable : ^INTEGER;
In order to use the pointer, you must allocate memory using the procedure NEW:
NEW(PtrVariable);
This assigns a memory address to the pointer variable PtrVariable (e.g., 709Ch). The value stored in this address is the actual data. To refer to the location pointed to by the pointer PtrVariable, use the variable name
PtrVariable^
which is treated like a regular variable. For example, you may assign it a numeric value,
PtrVariable^ := 500;
or assign it to another static variable
AnotherVariable := PtrVariable^;
This is demonstrated in the following diagram:
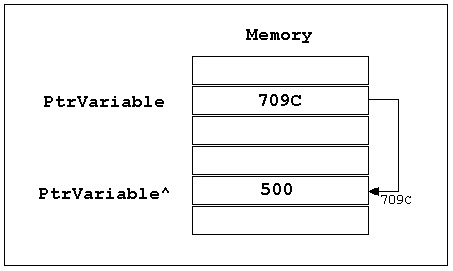
The memory allocated to the pointer may be released using the procedure DISPOSE:
DISPOSE(PtrVariable);
Once a pointer is disposed it becomes undefined.
In the following program, these features are demonstrated. An integer variable (MyInteger), and an integer pointer (MyIntegerPointer) are declared. The contents of the allocated location MyIntegerPointer^ are assigned to the variable MyInteger. Both, when printed, should give the same value (500).
{ ------------------------- figure 11-2 ------------------------------- }
PROGRAM PointerExample(OUTPUT);
VAR
MyIntegerPointer :^INTEGER;
MyInteger :INTEGER;
BEGIN
MyInteger := 50;
NEW(MyIntegerPointer);
MyIntegerPointer^ := 500;
MyInteger := MyIntegerPointer^;
WRITELN('The value of MyInteger is: ', MyInteger);
WRITELN('The value pointed to by MyIntegerPointer is: '
, MyIntegerPointer^);
DISPOSE(MyIntegerPointer);
WRITELN('Press any key to continue...');
READLN
END.
Notice that the procedure DISPOSE is not necessary in this program, because the memory is deallocated automatically when the program ends. It is used only for demonstration.
In the same way, you can declare pointers to other types; for example,
VAR
MyCharPointer :^CHAR;
MyStringPointer :^STRING;
MyRealPointer :^REAL;
Before you try to use any of these pointers, remember to use the procedure NEW to allocate memory for each one:
NEW(MyCharPointer);
NEW(MyStringPointer);
NEW(MyRealPointer);
When you are finished, you may use DISPOSE to deallocate memory associated with each of them:
DISPOSE(MyCharPointer);
DISPOSE(MyStringPointer);
DISPOSE(MyRealPointer);
|