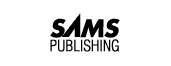
Teach Yourself COBOL in 21 days, Second Edition
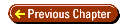
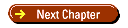
- D -
Handling Compiler Errors
One of the difficulties of COBOL is dealing with compiler error messages. COBOL
is very fussy about the syntax of the program. Some simple errors can produce problems
that are difficult to find.
Rules for Locating Errors
The following are two rules to remember at all times:
- Check for spelling errors. Check that you haven't misspelled IDENTIFICATION
DIVISION or any of the other divisions. Check that you haven't omitted or added
a period. Check that you haven't typed a zero when you meant to type the letter O.
- If the error that the compiler gives you doesn't seem to make sense after checking
the line that contains the error, look earlier in the code for an error.
General Problem Areas
The following are general procedural and typing errors to watch for and avoid:
- Misspelling the name of a DIVISION. In Micro Focus Personal COBOL, misspelling
IDENTIFICATION DIVISION produces an error of PROCEDURE DIVISION MISSING.
This is a devil to locate because everything around PROCEDURE DIVISION might
look good. You have to go all the way back to the top of the program to locate the
error.
- Misspelling the name of a required SECTION.
- Misspelling the name of any required PARAGRAPH.
- Starting a DIVISION, SECTION, or PARAGRAPH outside
of Area A. Area A is columns 8-11.
- Leaving a period off the end of a DIVISION, SECTION, or PARAGRAPH.
- Adding an unnecessary hyphen to a DIVISION or SECTION name.
For example, IDENTIFICATION-DIVISION or WORKING-STORAGE-SECTION
is wrong.
Specific Errors for Different Compilers
The following is a list of some errors that are hard to catch:
- Any type of error with a whole DIVISION. For example, the compiler might
print a message such as the following:
- MISSING PROCEDURE DIVISION
- or
- Expected DATA DIVISION but found something else.
- If the DIVISION is in the program and seems to be okay, the error is
in an earlier DIVISION. Check the spelling and punctuation.
- An unexpected end-of-file error message. This error can happen with some compilers
when you omit the period from the last statement in the program.
- The compiler error indicates that a paragraph name or data name is invalid, but
when you inspect the name there doesn't seem to be anything wrong with it.
- This error can be caused by using the same name for a paragraph and a variable.
For example, this type of error can occur if you have a variable with a name such
as
01 SAVE-THE-TOTAL PIC 9999.
- and in the program you have a paragraph named the following:
SAVE-THE-TOTAL.
PERFORM DO-SOMETHING.
- When this occurs, compilers do not always provide a clear description of the
error. Paragraph and data names should be unique.
- The compiler produces an error indicating that a variable name is ambiguous,
such as the following:
MOVE 12 TO ANOTHER-NUMBER
Error: Destination is ambiguous
- This error is caused by having two different variables with the same name. There
is another way to get around this problem, but it is much simpler if every variable
has a unique name. Bonus Day 2, "Miscellaneous COBOL Syntax," covers the
use of qualified data names and explains how you can use variables with the same
name when it is necessary.
- One common error in COBOL is accidentally typing a 0 (zero) when you
intended an O (uppercase letter O). For example, the following might cause
the compiler to generate strange errors:
MOVE 10 T0 THE-NUMBER
- The error might look like the following:
OPERAND not declared.
- Upon inspection, THE-NUMBER is found to be declared, so what's the problem?
On closer inspection, the T0 is spelled with a zero, so the compiler error
message just confuses the issue.
- The program seems to keep running when it should have stopped.
- This problem is produced by a combination of errors. First, the compiler usually
is one that does not care about sentences and paragraphs starting in the correct
positions. Second, the command to stop the program, STOP RUN, has been typed
incorrectly as STOP-RUN, with a hyphen.
- When the compiler sees something like the following, it does not see this as
a paragraph containing the sentence STOP RUN:
005500 PROGRAM-DONE.
005600 STOP-RUN.\
- Instead, it sees it as a paragraph named PROGRAM-DONE containing no
sentences and a paragraph named STOP-RUN containing no sentences.
- An unusual problem that produces oddball compiler errors is surprising reserved
words. I ran into one while writing this book. In one program, I had created variables
named DISPLAY-1, DISPLAY-2, DISPLAY-3, and DISPLAY-4.
This program compiled and ran fine under ACUCOBOL, but produced a very strange error
when compiled under Micro Focus Personal COBOL. It turned out that DISPLAY-1
is a reserved word in Micro Focus Personal COBOL, and its use as a variable name
made the compiler a bit nuts.
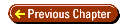
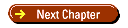
© Copyright, Macmillan Computer Publishing. All
rights reserved.